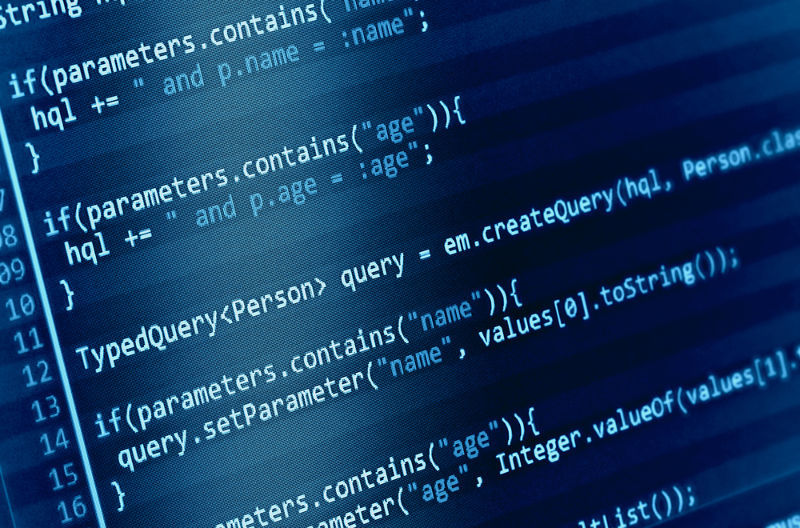
//MAIN CLASS
package alıştırmalar;
import java.util.*;
public class exercise12_4 {
public static void main(String[] args) {
// TODO Auto-generated method stub
boolean coninue=true;
while(coninue){
try {
calis();
} catch (IllegalArgumentException ex) {
System.out
.println("input must be positive!! please reenter the input \n");
}
catch(InputMismatchException e){
System.out.println("Program was terminated!");
coninue=false;
}
}
}
public static void calis() {
Scanner input = new Scanner(System.in);
System.out.println("\n---------INTEREST CALCULATOR----------");
System.out.println("enter annual ınterest rate : 8.25 e.g = ");
double annualInterestRate = input.nextDouble();
if (annualInterestRate <= 0)
throw new IllegalArgumentException();
System.out.println("enter number of years as ana integer ");
int numberOfYears = input.nextInt();
if (numberOfYears <= 0)
throw new IllegalArgumentException();
System.out.println("enter loan amount : 120000.95 e.g = ");
double loanAmount = input.nextDouble();
if (loanAmount <= 0)
throw new IllegalArgumentException();
Loan loan = new Loan(annualInterestRate, numberOfYears, loanAmount);
System.out.printf("the loan was created on %s\n"
+ "the montly payment is %.2f\nThe total payment is %.2f\n",
loan.getLoanDate().toString(), loan.getMonthlyPayment(),
loan.getTotalPayment());
}
}
//LOAN CLASS
package alıştırmalar;
public class Loan {
private double annualInterestRate;
private double loanAmount;
private int numberOfYears;
private java.util.Date loanDate;
public Loan(){
this(2.5,1,1000);
}
public Loan(double annualInterestRate,int numberOfYears,double loanAmount){
this.annualInterestRate=annualInterestRate;
this.numberOfYears=numberOfYears;
this.loanAmount=loanAmount;
loanDate=new java.util.Date();
}
public double getAnnualInterestRate(){
return annualInterestRate;
}
public void setAnnualInterestRate(double annualInterestRate){
this.annualInterestRate=annualInterestRate;
}
public int getNumberOfYears(){
return numberOfYears;
}
public void setNumberOfYears(int numberOfYears){
this.numberOfYears=numberOfYears;
}
public double getLoanAmount(){
return loanAmount;
}
public void setLoanAmount(double loanAmount){
this.loanAmount=loanAmount;
}
public double getMonthlyPayment(){
double monthlyInterestRate=annualInterestRate/1200;
double monthlyPayment=loanAmount*monthlyInterestRate/(1-(1/Math.pow(1+monthlyInterestRate,numberOfYears*12)));
return monthlyPayment;
}
public double getTotalPayment(){
double totalPayment=getMonthlyPayment()*numberOfYears*12;
return totalPayment;
}
public java.util.Date getLoanDate(){
return loanDate;
}
}