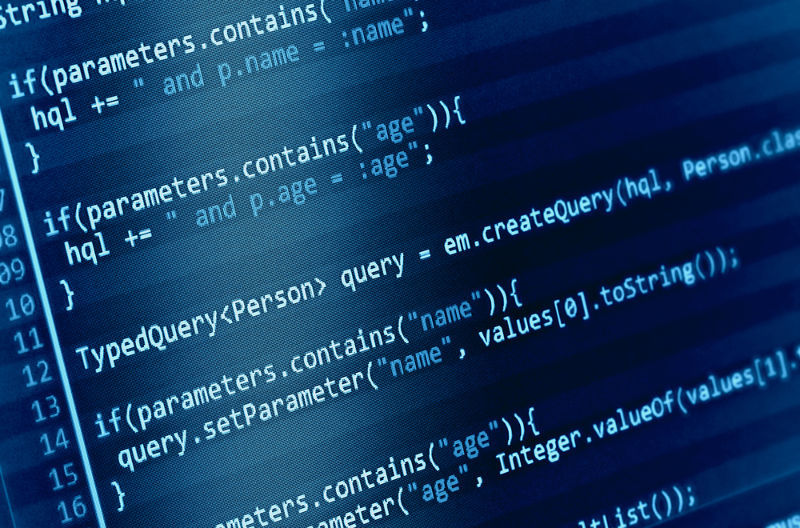
/*
Use the reverse method to implement isPalindrome. A number is a palindrome
if its reversal is the same as itself. Write a test program that prompts the
user to enter an integer and reports whether the integer is a palindrome.
*/
package Calismalarim;
import java.util.Scanner;
public class Palindrome_Integer {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("enter a number");
int number = input.nextInt();
if (isPalindrome(number))
System.out.println("the number is a palindrome");
else
System.out.println("the number is not a palindrome");
}
public static int reverse(int number) {
String rev = "";
while (number != 0) {
int revN = number % 10;
rev = rev + revN;
number = number / 10;
}
return Integer.parseInt(rev);
}
public static boolean isPalindrome(int number) {
if (reverse(number) == number)
return true;
else
return false;
}
}