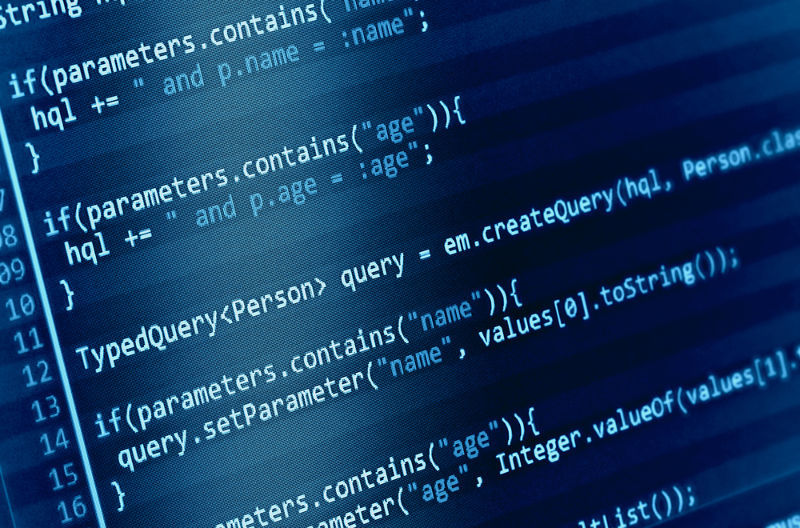
package ObjectOriantedHomeworks;
import java.util.*;
public class testHW2 {
public static void main(String[] args) {
// TODO Auto-generated method stub
Football_Player p1 = new Football_Player("Max", "FW", 1000000, 12);
Football_Player p2 = new Football_Player("JOSH", "DMF", 4000000, 22);
Football_Player p3 = new Football_Player("CASS", "OMF", 1000000, 12);
// NOT : DENEME AMAÇLI
/*
* Football_Team t = new Football_Team("WHITEAGLE", 2015, 4000000);
* t.addPlayer(p1); t.addPlayer(p2); t.addPlayer(p3);
* System.out.println(t.toString()); t.showMembers();
* t.removePlayer(p1); System.out.println(t.toString());
* t.showMembers();
*/
System.out.println(p1.toString());
System.out.println(p2.toString());
System.out.println(p3.toString());
Football_Team t = new Football_Team("WHITEAGLE", 2000, 4000000);
t.addPlayer(p1);
t.addPlayer(p2);
t.addPlayer(p3);
System.out.println(t.toString());
p3.setJerseyNumber(10);
t.addPlayer(p3);
System.out.println(t.toString());
p2.setPrice(500000);
t.addPlayer(p2);
System.out.println(t.toString());
String a = "2000 :Team has borned\n2002 :Became 3th in the legue\n2006 :Became champion of the Regional Legue";
String a3 = "2009 :Became 2nd of the 2nd Legue";
t.addAchivement(a);
t.addAchivement(a3);
System.out.println(t.toString());
}
}
class Football_Player {
private String name;
private String position;
private double price;
private int jerseyNumber;
private Date birthDate;
public Football_Player(String name, String pos, double price, int jN) {
this.name = name;
this.position = pos;
this.price = price;
this.jerseyNumber = jN;
birthDate = new Date();
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @param name
* the name to set
*/
public void setName(String name) {
this.name = name;
}
/**
* @return the position
*/
public String getPosition() {
return position;
}
/**
* @param position
* the position to set
*/
public void setPosition(String position) {
this.position = position;
}
/**
* @return the price
*/
public double getPrice() {
return price;
}
/**
* @param price
* the price to set
*/
public void setPrice(double price) {
if (price > (this.price / 10))
this.price = price;
else
System.out.println("!! Salary of player can't be 10% of previous Salary !!");
}
/**
* @return the jerseyNumber
*/
public int getJerseyNumber() {
return jerseyNumber;
}
/**
* @param jerseyNumber
* the jerseyNumber to set
*/
public void setJerseyNumber(int jerseyNumber) {
this.jerseyNumber = jerseyNumber;
}
/**
* @return the birthDate
*/
public Date getBirthDate() {
return birthDate;
}
/**
* @param birthDate
* the birthDate to set
*/
public void setBirthDate(Date birthDate) {
this.birthDate = birthDate;
}
public String toString() {
String info = "_________________________________________\n" + "\nName of the player : " + this.name
+ "\nPosition : " + this.position + "\nPrice : " + this.price + " $\nJersey number : "
+ this.jerseyNumber + "\nBirthdate : " + this.birthDate + "\n";
return info;
}
}
class Football_Team {
private String name;
private int foundationYear;
private double budget;
private String achivements[];
private Football_Player[] player;
public Football_Team(String name, int FYear, double budget) {
this.name = name;
foundationYear = FYear;
this.budget = budget;
achivements = new String[1];
player = new Football_Player[1];
}
public void addPlayer(Football_Player fp) {
boolean isSame = false;
int index = 0;
for (int i = 0; i < player.length; i++) {
if (player[0] == null)
break;
if (player[i].getJerseyNumber() == fp.getJerseyNumber()) {
isSame = true;
index = i;
break;
}
}
if (fp.getPrice() > this.budget) {
System.out.println("-----------------------------------------");
System.out.println("\n!! INSUFFICIENT BUDGET !!");
System.out.println(fp.getName() + " couldn't be joined into your team");
System.out.println("-----------------------------------------");
return;
}
if (isSame) {
System.out.println("-----------------------------------------");
System.out.println("\n!! JERSEY NUMBER HAS MATCHED !!");
System.out.println(fp.getName() + " and " + player[index].getName() + " have the same jersey number");
System.out.println("-Please rearrenge the JN of " + fp.getName() + " or " + player[index].getName() + "\n");
System.out.println("-----------------------------------------");
return;
}
System.out.println("-----------------------------------------");
System.out.println("!! SUCCESSFULLY TRANSFERED !!");
System.out.println(fp.getName() + " has been transfered");
System.out.println("-----------------------------------------");
if (player[0] != null) {
player = increaseSize();
int length = player.length;
player[--length] = fp;
} else
player[0] = fp;
this.budget = this.budget - fp.getPrice();
}
public void addAchivement(String a) {
if (achivements[0] != null) {
achivements = increaseAchevementSize();
int length = achivements.length;
achivements[--length] = a;
} else
achivements[0] = a;
}
private String[] increaseAchevementSize() {
String a2[];
int length = achivements.length;
a2 = new String[++length];
for (int i = 0; i < achivements.length; i++)
a2[i] = achivements[i];
return a2;
}
private Football_Player[] decreaseSize(int index) {
Football_Player fp2[] = new Football_Player[player.length - 1];
for (int i = 0, j = 0; i <= fp2.length; i++) {
if (i == index)
continue;
fp2[j] = player[i];
j++;
}
return fp2;
}
private Football_Player[] increaseSize() {
Football_Player fp2[];
int length = player.length;
fp2 = new Football_Player[++length];
for (int i = 0; i < player.length; i++)
fp2[i] = player[i];
return fp2;
}
public void removePlayer(Football_Player fp) {
for (int i = 0; i < player.length; i++) {
if (fp.getName().equals(player[i].getName())) {
player = decreaseSize(i);
break;
}
}
this.budget = this.budget + fp.getPrice();
}
public void showMembers() {
System.out.println("**** MEMBERS OF THE TEAM ****\n");
for (int i = 0; i < player.length; i++) {
System.out.println(player[i].toString());
}
}
public String toString() {
String info;
if (achivements[0] == null) {
info = "\n" + " TEAM INFOTMATION\n" + "_________________________________________" + "\nName of the Team :"
+ this.name + "\nFoundation Year : " + this.foundationYear + "\nBudget : " + this.budget
+ " $\nNumber of players : " + player.length + "\nAchivements :\nNONE"
+ "\n_________________________________________\n";
} else {
String info2 = "";
for (int i = 0; i < achivements.length; i++) {
info2 += "\n" + achivements[i];
}
info = "\n" + " TEAM INFOTMATION\n" + "_________________________________________"
+ "\nName of the Team :" + this.name + "\nFoundation Year : " + this.foundationYear + "\nBudget : "
+ this.budget + " $\nNumber of players : " + player.length + "\nAchivements :" + info2
+ "\n_________________________________________\n";
}
return info;
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @param name
* the name to set
*/
public void setName(String name) {
this.name = name;
}
/**
* @return the foundationYear
*/
public int getFoundationYear() {
return foundationYear;
}
/**
* @param foundationYear
* the foundationYear to set
*/
public void setFoundationYear(int foundationYear) {
this.foundationYear = foundationYear;
}
/**
* @return the budget
*/
public double getBudget() {
return budget;
}
/**
* @param budget
* the budget to set
*/
public void setBudget(double budget) {
this.budget = budget;
}
}