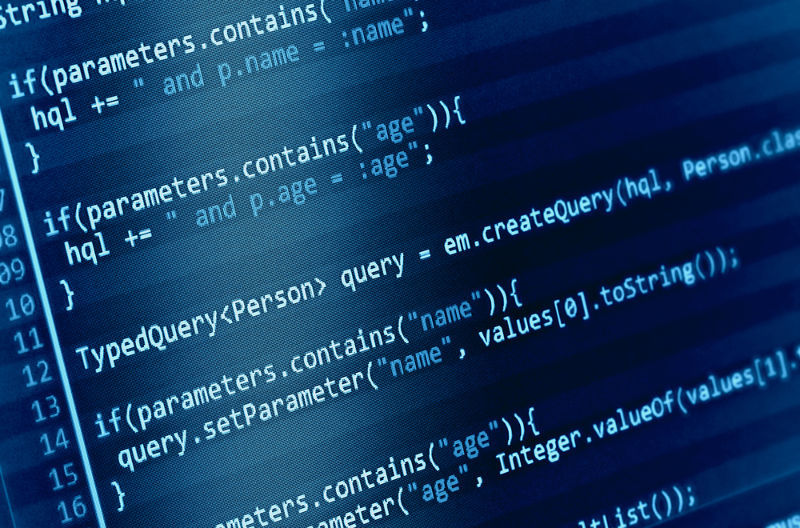
package Classes;
import java.util.*;
public class AtmMachine_2 {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner input = new Scanner(System.in);
Account[] account = new Account[10];
int id;
byte choose;
for (int i = 0; i < account.length; i++)
account[i] = new Account(i);
System.out.println("enter an id :");
id = input.nextInt();
while (true) {
System.out.println("enter your decision\n--------------------");
menu();
choose = input.nextByte();
switch (choose) {
case 1:
System.out.println("--------------------\nenter a quantity");
account[id].setWithdraw(input.nextDouble());
break;
case 2:
System.out.println("--------------------\nenter a quantity");
account[id].setDeposite(input.nextDouble());
break;
case 3:
System.out.println("current account: " + account[id].getAccount());
break;
case 4:
System.out.println("enter an id :");
id = input.nextInt();
break;
default:
System.out.println("wrong choise!!would you like to perform another process?");
}
}
}
public static void menu() {
String s="1)withdraw\n"
+ "2)deposite\n"
+ "3)see account\n"
+ "4)exit";
System.out.println(s);
}
}
class Account {
private double deposite;
private double withdraw;
private double account;
private int id;
public Account(int id) {
this.id = id;
account = 100;
deposite = 0;
withdraw = 0;
}
public double getDeposite() {
return deposite;
}
public void setDeposite(double deposite) {
this.deposite = deposite;
account += deposite;
}
public double getWithdraw() {
return withdraw;
}
public void setWithdraw(double withdraw) {
this.withdraw = withdraw;
if (account >= -500 && (account - withdraw) >= -500)
account -= withdraw;
else
System.out.println("Sorry restricted account!");
}
public double getAccount() {
return account;
}
}